Update Cart Drawer Dawn theme after adding product throught add.js
One of the key features of an optimized Shopify store is a smooth shopping experience. When a customer adds a product to the cart, they expect an instant update in the cart drawer without needing a page refresh. By default, Shopify’s Dawn theme doesn’t refresh the cart drawer automatically, but you can modify it using JavaScript.
In this guide, we’ll show you two methods to update the cart drawer dynamically after adding a product.
Method 1: Using AJAX and Fetch API
This method involves using an AJAX request to add a product and then fetching the updated cart drawer HTML.
Steps to Implement:
- Make an AJAX call to add the product to the cart.
- Fetch the updated cart drawer section.
- Replace the old cart drawer content with the updated one.
Code Implementation:
$.ajax({
type: 'POST',
url: '/cart/add.js',
data: JSON.stringify(data),
dataType: 'json',
contentType: 'application/json',
success: function(response) {
console.log('Product added to cart:', response);
fetch(`${routes.cart_url}?section_id=cart-drawer`)
.then((response) => response.text())
.then((responseText) => {
const html = new DOMParser().parseFromString(responseText, 'text/html');
const selectors = ['cart-drawer-items', '.cart-drawer__footer'];
for (const selector of selectors) {
const targetElement = document.querySelector(selector);
const sourceElement = html.querySelector(selector);
if (targetElement && sourceElement) {
targetElement.replaceWith(sourceElement);
}
}
})
.catch((e) => {
console.error(e);
});
},
error: function(XMLHttpRequest, textStatus) {
console.error('Error adding product to cart:', textStatus);
}
});
This script ensures that the cart drawer updates seamlessly whenever a product is added.
Method 2: Modifying cart-drawer.js
Another efficient approach is to modify the cart-drawer.js
file and use the refresh()
function to reload the cart drawer.
Steps to Implement:
- Add the following function to
cart-drawer.js
:
async refresh(dontRefreshCartItems) {
try {
const response = this.getSectionsToRender().map((section) => section.id);
const cartResponse = await fetch(`?sections=${response.join(',')}`);
const sections = await cartResponse.json();
let ADDTOCART_OBJECT = { sections: sections }
this.renderContents(ADDTOCART_OBJECT);
if (this.classList.contains('is-empty')) this.classList.remove('is-empty');
} catch (error) {
console.log(error); // eslint-disable-line
this.dispatchEvent(new CustomEvent('on:cart:error', {
bubbles: true,
detail: {
error: this.errorMsg.textContent
}
}));
}
}
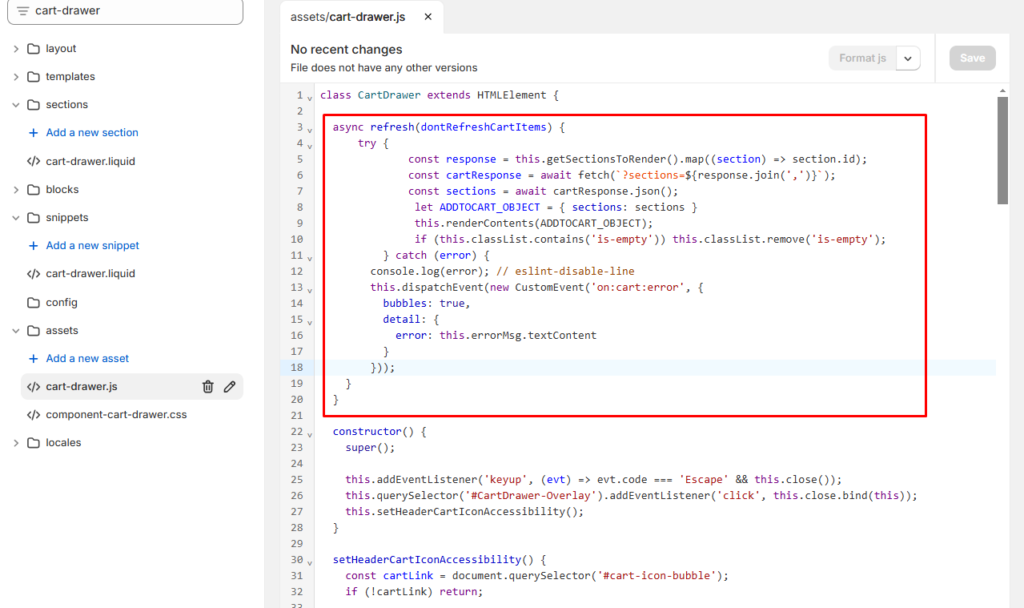
- Modify the product add-to-cart function to call the
refresh()
function after adding a product:const this_cartDrawer = document.querySelector('cart-drawer'); this_cartDrawer.refresh(true);
Fetch API Using refresh() function:
const data = {
id: '50324841038137', // Replace with your product ID
quantity: 1
};
fetch('/cart/add.js', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(data)
})
.then(response => response.json())
.then(response => {
const this_cartDrawer = document.querySelector('cart-drawer');
this_cartDrawer.refresh(true);
})
.catch(error => {
console.error('Error adding product to cart:', error);
});
This ensures that whenever a product is added, the cart drawer refreshes itself dynamically.
Final Thoughts
Both methods work well for updating the cart drawer without requiring a page reload. The first method is simpler and works with minimal code changes, while the second method offers better flexibility by integrating directly into cart-drawer.js
.
If you’re using Shopify’s Dawn theme and want to improve the user experience, implementing one of these methods will significantly enhance the cart update functionality.
Try them out and let us know which method worked best for you!
Need Help?
If you’re facing issues implementing these solutions or need custom Shopify development, feel free to reach out. We’d love to assist you!